Getting Started with NSLayoutAnchor
April 9, 2016
A newer version of this post is available called Getting Started with NSLayoutAnchor (Swift 3.1)
One of the most challenge parts of creating any app is figuring out how you're gonna lay out the views on a screen. With the addition of more screen sizes it's not getting any easier but thankfully apple is also offering us more ways to work with auto layout. Here I'm gonna take an introductory look at the new API introduced in iOS 9 NSLayoutAnchor. I'll show you it's improvements over NSLayoutConstraints and how to use them in your app.
The first thing you probably noticed when looking for ways to create constraints is ALL of the ways to do it! You can use interface builder, stack views, visual format, and last but not least anchors. There's no one perfect way to do this but I'd argue the one with the current best readability is NSLayoutAnchor class. If you're creating constraints in code you'll probably use either NSLayoutConstraints or NSLayoutAnchor so here's what the two look like in code.
I start off by creating an array of NSLayoutConstraint so I can activate them all together. More importantly though the LayoutConstraints look like it just drove up in a white van trying to sell homemade candy, it's scary. My Anchors look rather charming though because it's much easier to just see exactly what they're doing.
Both my set of constraints for NSLayoutConstraint and NSLayoutAnchor above accomplish the exact same task of being constraints.
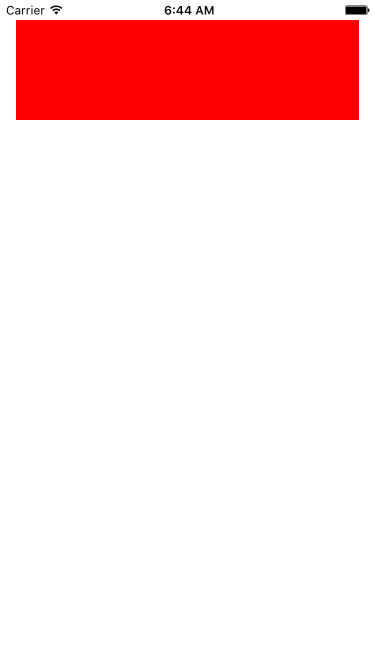
Readability
When you look at each type on constraint which one is more readable to you? Somehow NSLayoutAnchor manages to require less code and do just as much. I can even do better than I did above if I create one little variable.
It's an easy fix to already readable code but now it's impossible to not see it's advantages over NSLayoutConstraint. There is one less obvious advantage though.
Type Checking
In the above code I've clumsily tried anchoring myView's height equal to the super views bottom anchor. I'm getting an error before I even compile saying NSLayoutYAxisAnchor is not the expected type (It's looking for a CGFloat). If I replicated the mistake with NSLayoutConstraint I don't get a warning before I compile
NSLayoutAnchor has three subclasses (NSLayoutXAxisAnchor, NSLayoutYAxisAnchor,NSLayoutDimension) that provide some helpful type checking but no additional methods. This will still allow mistakes if you provide a parameter of the same class but it's more helpful than LayoutConstraints are.
Creating NSLayoutAnchors
First you'll need your object to constrain.
Next, I'll systematically constrain my view using the normal points such as topAnchor, leadingAnchor, trailingAnchor, or bottomAnchor depending on what I'd like to do with my view. There are TONS of other options too such as centerXAnchor, firstBaseLineAnchor, etc...
I'll then decide how I would like it to be constrained to an object. In the above I used the method constraintEqualToAnchor(_: ) but there's plenty more such as constraintGreaterThanOrEqualToAnchor(_: ), constraintLessThanOrEqualToAnchor(_: ) and a lot more to cover all the possible ways you'd like to constrain your objects.
Lastly, you'll need to pick another object's anchor such as a super view, another child view, or the layoutGuide and activate it by setting the property 'active' to true.
That sums it up for the introduction to NSLayoutAnchor. I'll be diving in deeper to more complicated layouts next week and how to use priorities along with this new API. If this was helpful to you please leave a comment and let me know how share it with a fellow learner! :) Enjoy! Auf wiedersehen!